Query Expressions
Query expressions provide an elegant and powerful way to retrieve the members of a list filtered by certain conditions, ordered in a certain direction, and/or processed by certain calculations.
The result of a query expression is a new list, which, itself, can be used wherever any list can be used.
General form
The general form of a query expression is as follows. The square brackets are not part of the syntax, but indicate those parts that are optional.
from variable in sourceList [ where expression ] [ orderby expression(s) ] [ distinct ] [ reverse ] [ skip count] [ take count ] [ select expression ]
Where:
- variable is the name of a variable called the iteration variable, which represents each successive member of the source list. We can pick any name we want; the variable is created spontaneously, and is local to the query expression.
- sourceList is any expression that evaluates to a list. A query expression does not change the contents of its source list.
- where expression is an optional clause that limits the resultant list to only those members for which the specified expression evaluates to true.
- orderby expression(s) is an optional clause that sorts the resultant list, based on the value(s) of the specified expression(s). Multiple expressions, which represent multiple levels of sorting, are separated by commas. Any expression may be followed by the keyword descending, which reverses the direction of the sort at that level.
- distinct is an optional clause that removes any duplicate entries from the resultant list.
- reverse is an optional clause that reverses the order of the resultant list.
- skip count is an optional clause that removes a certain number of items from the beginning of the resultant list. The count is any expression that resolves to a number.
- take count is an optional clause that limits the resultant list to a certain number of items, starting from the beginning of the list. The count is any expression that resolves to a number.
- select expression is an optional clause that specifies how each member of the resultant list should be calculated. If this clause is omitted, the resultant list is composed of successive values of the iteration variable (var).
Aside from the initial from clause and the (optional) final select clause, there may be any number of each clause type, and they may appear in any sequence. The clauses are applied in the sequence in which they appear.
Like everything else in GCScript, a query expression may be written on one line or across multiple lines. Multiple lines are often preferred because they are easier to read. However, either of these forms is equally acceptable:
from ln in line01 where ln.Length <= maxLength orderby ln.StartPoint.X select ln.StartPoint.X
from ln in line01 where ln.Length <= maxLength orderby ln.StartPoint.X select ln.StartPoint.X
What can we do with the result of a query expression?
Well, of course, the answer is, anything we want. For example, we can...
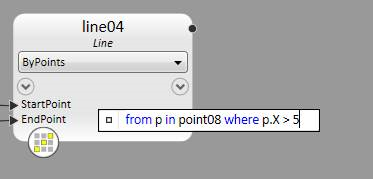
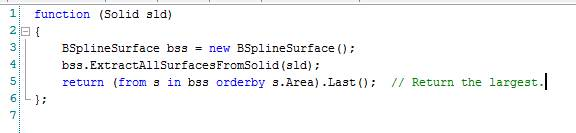
BSplineSurface largest = (from s in bss orderby s.Area).Last(); // Do further processing with 'largest'...
- Use it in the context of a foreach statement. For more information, see .
Specifying the type of the iteration variable
It is possible to explicitly specify the type of the iteration variable, e.g:
from Line ln in line01 where ln.Length <= maxLength
The primary advantage of doing so is that, subsequently, the script editor with produce a proper auto-completion list for the iteration variable.
The foreach statement
Most of the query expression clauses are also available in the foreach statement